Testing machine learning code is essential for ensuring the quality and performance of your models. However, it can be challenging due to complex data, algorithms, and frameworks.
Unit tests isolate and test individual functions or modules, allowing you to identify and fix bugs early in the development process. Here’s a breakdown of key concepts:
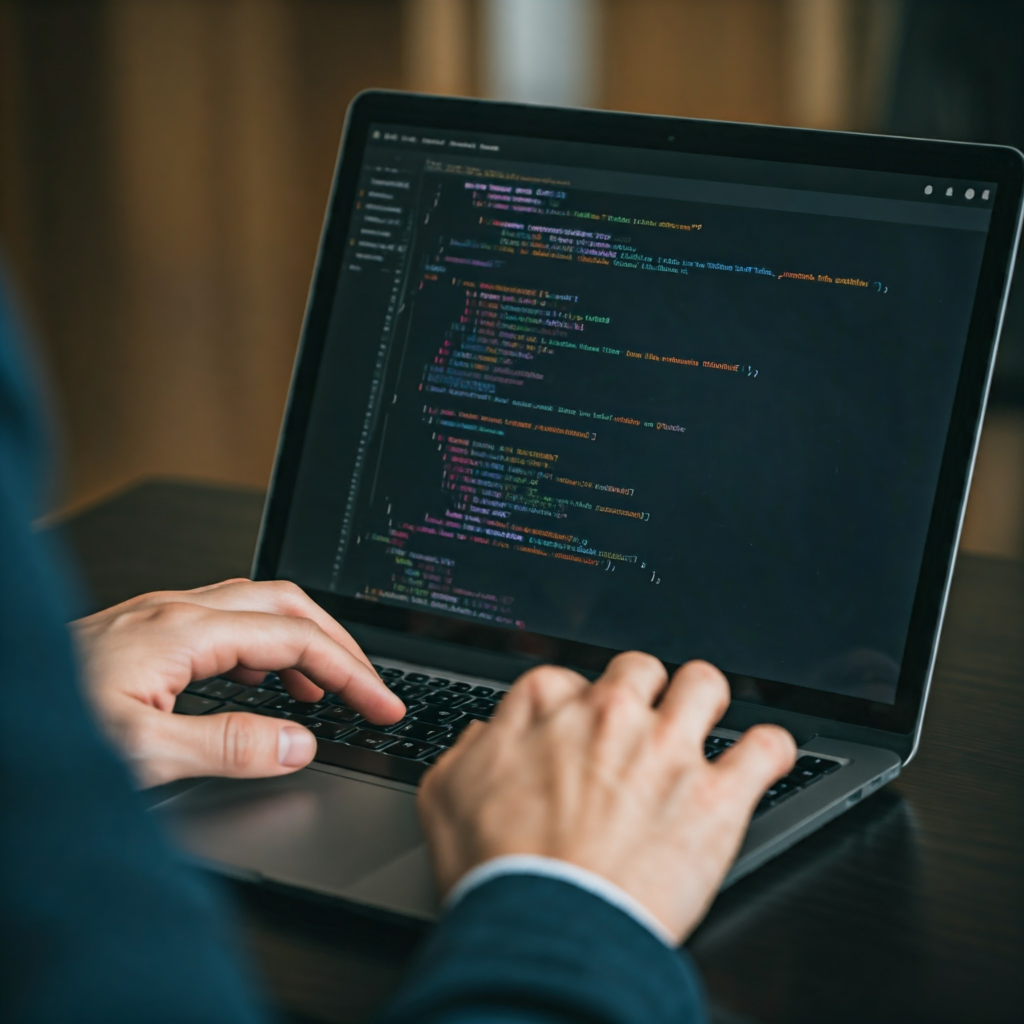
- Why Unit Test Machine Learning Code? Machine learning code often involves complex algorithms and data manipulation. Unit tests help to:
- Improve code maintainability by making it easier to understand and modify.
- Increase confidence in the correctness of individual code components.
- Catch errors early in the development cycle.
- What to Unit Test? Focus on testing:
- Data preprocessing functions (e.g., scaling, normalization).
- Feature engineering functions.
- Individual model components (e.g., layers in a neural network).
- Custom helper functions used throughout your code.
- Challenges of Unit Testing Machine Learning Code
- Non-deterministic behavior: Some machine learning algorithms have elements of randomness, making it trickier to write deterministic unit tests.
- Data dependency: Unit tests often rely on specific data inputs, requiring careful consideration of mock or test data generation.
- Best Practices for Unit Testing Machine Learning Code
- Use a mocking framework to isolate functions from external dependencies like data sources.
- Write clear and concise test cases that document the expected behavior.
- Leverage testing frameworks like pytest or unittest that are familiar to many developers.
- Consider using a test-driven development (TDD) approach, where you write the test cases before the actual code.
- Choose the Right Framework:
- Select a framework (TensorFlow, PyTorch, Scikit-learn) that aligns with your project needs. Consider factors like ease of use, community support, and scalability. Maintain consistency to avoid confusion.
- Write Unit Tests:
- Create small code snippets to test individual functions or components using libraries like pytest or unittest.
- Follow Test-Driven Development (TDD) by writing tests before the code to ensure they initially fail and pass only when functional.
- Leverage Mock Data:
- Utilize mock data (fake or simulated) to test specific functionalities without relying on real data. Tools like Faker or libraries like NumPy and Pandas can help create mock data.
- Ensure mock data replicates the structure, type, and distribution of your real data, covering various scenarios and edge cases.
- Perform Integration Tests:
- These tests verify how different parts of your code work together. Use frameworks like pytest or unittest to conduct these tests after completing unit tests.
- Automate integration tests using Continuous Integration (CI) tools like GitHub Actions to run them with every code change.
- Monitor and Debug Your Models:
- Monitor and debug models to improve performance and accuracy. Monitoring involves collecting metrics and logs to assess training, validation, testing, and deployment. Debugging identifies and fixes issues like overfitting, underfitting, and bias.
- Utilize tools like TensorBoard, MLflow, or Weights and Biases for monitoring, debugging, and tracking experiments.
- Review and Refactor Your Code:
- Regularly review your code for errors, inconsistencies, and bad practices. Consider seeking feedback from other developers.
- Refactor your code to enhance clarity, conciseness, and modularity. Use tools like pylint or code formatters like black to enforce consistent style and conventions.
Writing unit tests is a crucial step in ensuring the robustness and reliability of your machine learning code.
By incorporating unit testing into your machine learning development process, you can significantly improve the quality and reliability of your code.